Step 1: Create the new cs file called isvalidAgeAttribute.cs
Step 2: Copy the below code into the isvalidAgeAttribute.cs file
public class isvalidAgeAttribute : ValidationAttribute,IClientValidatable
{
private string _empAge;
public override bool IsValid(object value)
{
if (value != null)
{
_empAge = value.ToString();
_empAge = _empAge.Trim();
Regex regex = new Regex(@"^[0-9]+$");
return regex.IsMatch(_empAge);
}
return true;
}
public IEnumerable<ModelClientValidationRule> GetClientValidationRules(ModelMetadata metadata,ControllerContext context)
{
ModelClientValidationRule newRule = new ModelClientValidationRule();
newRule.ErrorMessage = "Please type your valid age";
//validationparameters name and validationType name should be small case
newRule.ValidationParameters.Add("isvalidage", _empAge);
newRule.ValidationType = "agevalidation";
yield return newRule;
}
}
Now use the custom validation in your model class, just import the namespace and add the isvalidAge attribute in the age property of employee model as shown.
EmployeeModel.cs
namespace MVC4Examplesbygiri.Models
{
public class EmployeModel
{
public string firstName { get; set; }
public string lastName { get; set; }
[isvalidAge(ErrorMessage="Please type your valid age")]
public string Age { get; set; }
public decimal Salary { get; set; }
}
}
In the client side as shown below, you have to create adapter and validator. To create adapter you have to use addSingleVal method as shwon below. The purpose of adapter is to get the meta-data from the render view and pass it to the validator object for validation. The validator object used one method called addMethod for validation as shown below.
View:-
@{
ViewBag.Title = "customclientValidation";
}
<h2>customclientValidation</h2>
@model MVC4Examplesbygiri.Models.EmployeModel
@*
//for client validation add the following scripts from the scripts folder
*@
@section clientvalidScripts
{
<script src="~/Scripts/jquery-1.8.2.min.js"></script>
<script src="~/Scripts/jquery.validate.min.js"></script>
<script src="~/Scripts/jquery.validate.unobtrusive.min.js"></script>
}
<script type="text/javascript">
//step 1:- create an adapter
//first parameter -> reference to ValidationType property name set on the server side
//second parameter -> reference to ValidationParameters name set on the server side
$.validator.unobtrusive.adapters.addSingleVal("agevalidation", "isvalidage");
//step 2:- create a validator
//first parameter -> reference to validationType property name set on the server side
//second parameter ->a function to invoke when validation occurrs
$.validator.addMethod("agevalidation", function (value, element, ageValidation) {
var age = value;
if (age.length > 0) {
var regex = new RegExp('^[0-9]+$', 'g');
if (age.match(regex))
return true;
else
return false;
}
else
return true;
});
</script>
@using (Html.BeginForm("customclientValidation", "Home", FormMethod.Post))
{
<table>
<tbody>
<tr>
<td>
@Html.LabelFor(m => m.firstName)
</td>
<td>
@Html.TextBoxFor(m => m.firstName)
</td>
</tr>
<tr>
<td>
@Html.LabelFor(m => m.lastName)
</td>
<td>
@Html.TextBoxFor(m => m.lastName)
</td>
</tr>
<tr>
<td>
@Html.LabelFor(m => m.Age)
</td>
<td>
@Html.TextBoxFor(m => m.Age)
@Html.ValidationMessageFor(m => m.Age)
</td>
</tr>
<tr>
<td>
@Html.LabelFor(m => m.Salary)
</td>
<td>
@Html.TextBoxFor(m => m.Salary)
</td>
</tr>
<tr>
<td></td>
<td>
<input type="submit" id="btn_submit" value="Add Employee" />
</td>
</tr>
</tbody>
</table>
}
Controller:-
public ActionResult customclientValidation()
{
return View();
}
[HttpPost]
public ActionResult customclientValidation(EmployeModel obj)
{
if (ModelState.IsValid)
{
//write your code
//redirect to confirmation page
}
//redisplay with errors
return View();
}
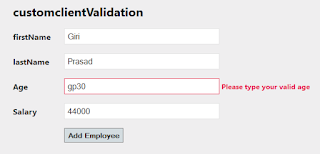
No comments:
Post a Comment